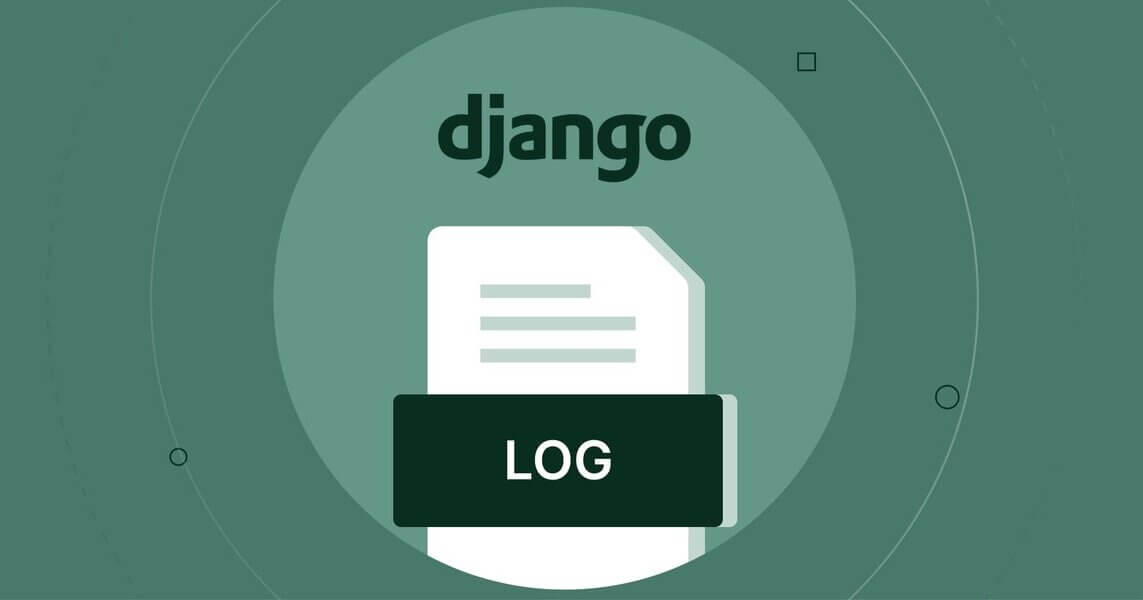
What is logging?
Logging in a software application refers to the process of recording events, activities, or messages that occur during
the execution of the application. These events are typically stored in log files or sent to a centralized logging system
for monitoring, analysis, debugging, or auditing purposes.
Importance of logging
- Recording Events: Logging captures various types of events that occur within an application, such as errors, warnings, informational messages, debug output, user actions, system events, and performance metrics.
- Debugging and Troubleshooting: Logs are crucial for debugging and troubleshooting issues in an application. Developers can examine log messages to identify and diagnose problems, trace the flow of execution, and understand the sequence of events leading up to an error or unexpected behavior.
- Monitoring and Alerting: Logs provide valuable insights into the health and performance of an application. Monitoring systems can analyze log data in real-time to detect anomalies, track trends, and trigger alerts based on predefined criteria, such as error rates, response times, or resource utilization.
- Auditing and Compliance: Logging helps in auditing and compliance efforts by maintaining a record of user actions, system activities, and security-related events. Log data can be used to track changes, investigate security incidents, and demonstrate regulatory compliance.
- Performance Analysis: Logging can also be used for performance analysis and optimization. By logging performance metrics such as response times, database queries, and resource usage, developers can identify bottlenecks, optimize code, and improve overall system efficiency.
- Historical Record: Logs serve as a historical record of what happened in an application over time. They can be valuable for historical analysis, forensic investigations, and post-mortem reviews of incidents or outages.
- Log Levels: Logging typically supports different levels of verbosity or severity, such as DEBUG, INFO, WARNING, ERROR, and CRITICAL. Developers can use these levels to control the amount of detail logged and prioritize messages based on their importance.
Django logging
Django logging allows you to capture and manage log messages generated by your Django application. It's a powerful tool
for debugging, monitoring, and analyzing the behavior of your application. Here's a breakdown of how Django logging work
Breakdown on Django logging
- Configuration: Django's logging system is configured in your project's settings.py file.
- Handlers: Handlers define where log messages are sent. Django provides various built-in handler classes like StreamHandler (outputs to console), FileHandler (writes to a file), and MailHandler (sends emails).
- Loggers: Loggers are responsible for emitting log messages. Each logger has a name, and you can define different loggers for different parts of your application.
- Log Levels: Log levels determine the severity of a log message. Django supports standard log levels like DEBUG, INFO, WARNING, ERROR, and CRITICAL.
- Formatters: Formatters define how log messages are formatted before they're emitted by a handler.
- Filters: Filters allow you to selectively suppress or modify log messages based on specific criteria.
- Usage: Once logging is configured, you can use the Python logging module to emit log messages from your Django application.
Details on breakdown:
Configuration:
Django's logging settings are typically configured in your project's settings.py file. You can specify things like
log format, handlers, and log levels here.
LOGGING = {
'version': 1,
'disable_existing_loggers': False,
'formatters': {
'verbose': {
'format': '{levelname} {asctime} {module} {message}',
'style': '{',
},
'simple': {
'format': '{levelname} {message}',
'style': '{',
},
},
'handlers': {
'console': {
'level': 'DEBUG',
'class': 'logging.StreamHandler',
'formatter': 'simple'
},
'file': {
'level': 'DEBUG',
'class': 'logging.FileHandler',
'filename': 'django_debug.log',
'formatter': 'verbose'
},
},
'loggers': {
'django': {
'handlers': ['console', 'file'],
'level': 'DEBUG',
'propagate': True,
},
'django.request': {
'handlers': ['file'],
'level': 'ERROR',
'propagate': False,
},
'myapp': {
'handlers': ['console', 'file'],
'level': 'DEBUG',
'propagate': False,
},
},
}
In this example, we configure a simple console handler for logging messages at the DEBUG level.
Explanation:
- version: Indicates the version of the logging configuration schema (always 1).
- disable_existing_loggers: When set to False, keeps existing loggers active.
- formatters: Define the format of log messages. In this example, two formats are defined: verbose and simple.
- handlers: Define how log messages are handled. The example shows two handlers: one for the console and one for a file.
- loggers: Define the loggers. The example includes the default django logger, a logger for django.request to handle request errors, and a custom logger for myapp.
Logging in Your Code:
You can use Python's built-in logging module to log messages from your Django application.
import logging
logger = logging.getLogger(__name__)
def my_view(request):
logger.debug('This is a debug message')
logger.info('This is an info message')
logger.warning('This is a warning message')
logger.error('This is an error message')
logger.critical('This is a critical message')
Here, we create a logger instance specific to the module where the logging occurs. This helps you to organize your logs better.
Log Levels:
Django supports several log levels, including DEBUG, INFO, WARNING, ERROR, and CRITICAL. You can specify the desired log
level for different parts of your application in the logging configuration.
- DEBUG: Detailed information, typically of interest only when diagnosing problems.
- INFO: Confirmation that things are working as expected.
- WARNING: An indication that something unexpected happened or indicative of some problem in the near future (e.g., ‘disk space low’). The software is still working as expected.
- ERROR: Due to a more serious problem, the software has not been able to perform some function.
- CRITICAL: A very serious error, indicating that the program itself may be unable to continue running.